Advanced CUDA examples
Overview
Teaching: 10 min
Exercises: 0 minQuestions
How do I find a suitable CUDA example to start from?
Objectives
Preview several CUDA examples
Understanding your NVIDIA GPU limitations
Visual Studio offers many ways to make use of the compute capability in your GPU. They provide several example projects so that users copy and modify working code to suite their needs. Some examples include:
- Simple useful examples (clock timings)
- Graphic examples (Mandelbrot)
- Imaging examples (denoising image)
- Simulation examples (smoke particles)
- Other examples
Simple examples
A simple example that focusing on measure execution time is the clock project. It runs a standard parallel reduction and uses a timer function to measure the amount of time spent in each kernel on the GPU.
As an exercise, you can change the number of blocks and threads to see how the GFLOPs increase until you reach the GPU limits. It is good to note what happens when the number of blocks/threads increases past the GPU limits. NOTE: It may not be what you expect.
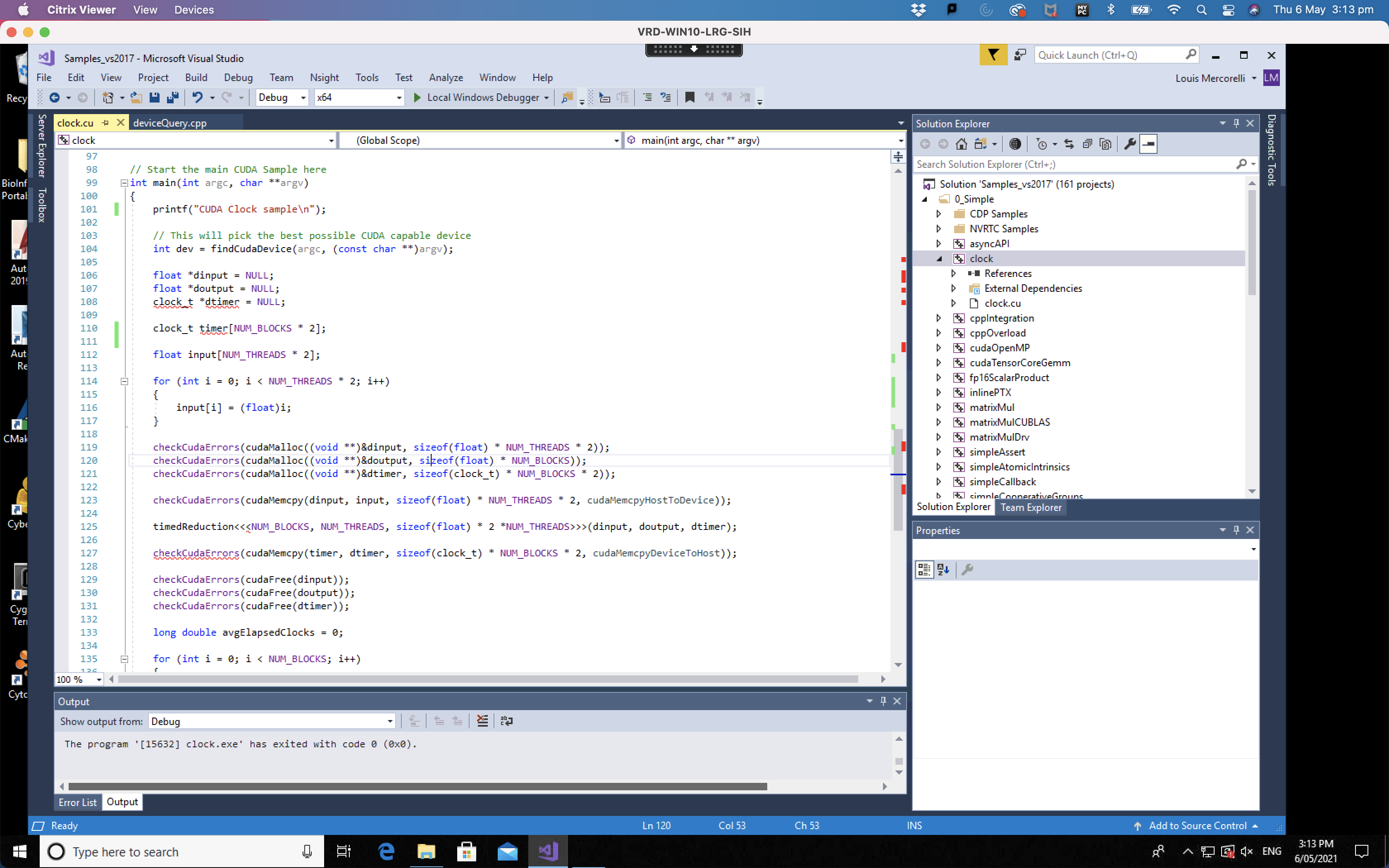
If you get stuck, look for the code below in the clock.cpp module and change the blocks and/or threads to help you to get started.
#define NUM_BLOCKS 64
#define NUM_THREADS 256
There are over 50 simple examples in the “0_Simple” folder. Two of the better examples are in the “CDP Sample” folder. The first is the cdpSimplePrint which highlights cuda dynamic parallelism using simple print statements to show how threads and blocks operate together on the GPU. The second is the “cdpSimpleQuickSort” which shows how to do a quick sort. A nice example that every computer science major would know from their first comp sci course.
Graphic examples
The Mandelbrot solution in the “2_Graphics” folder is a good example that is visually interesting.
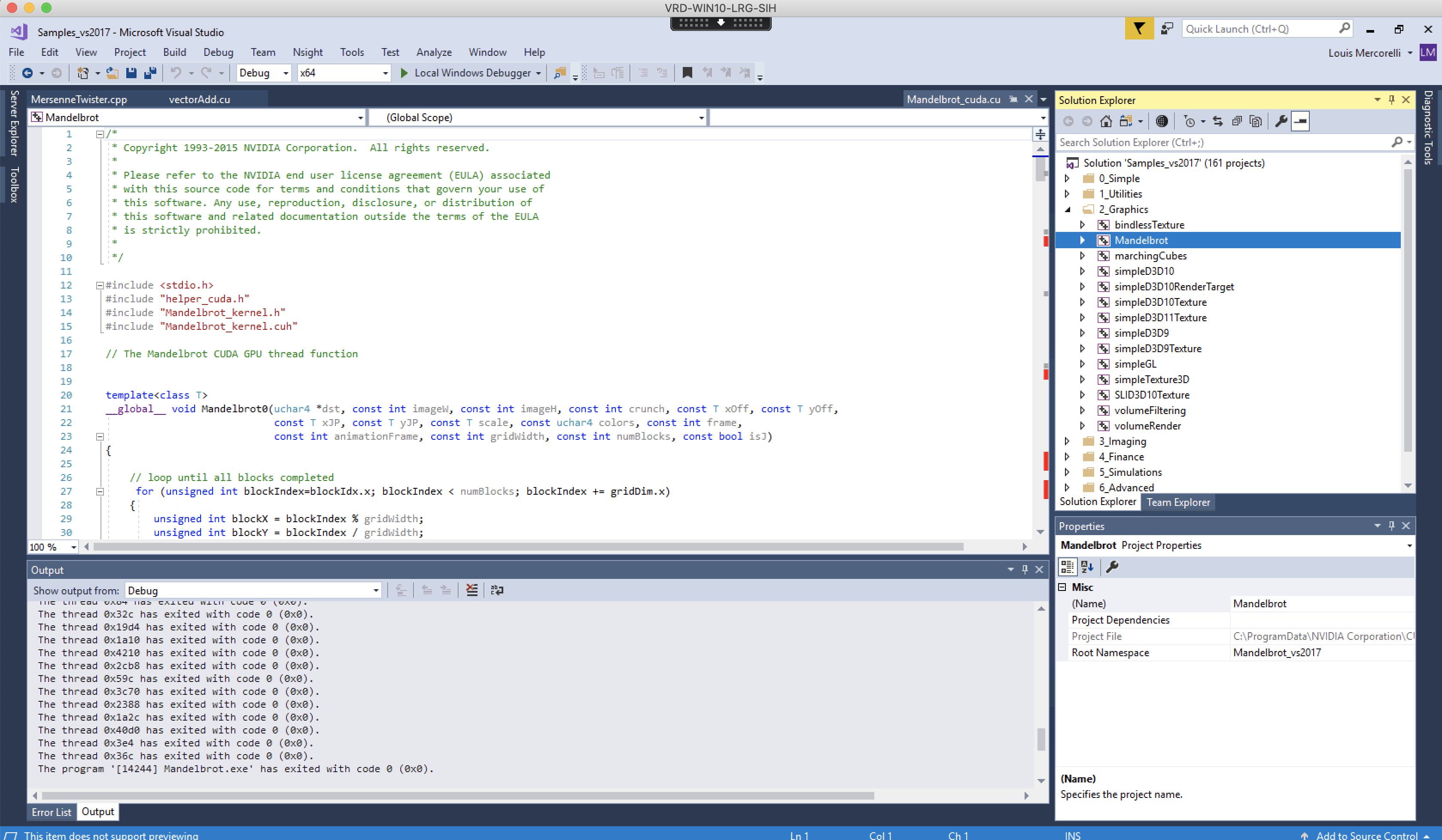
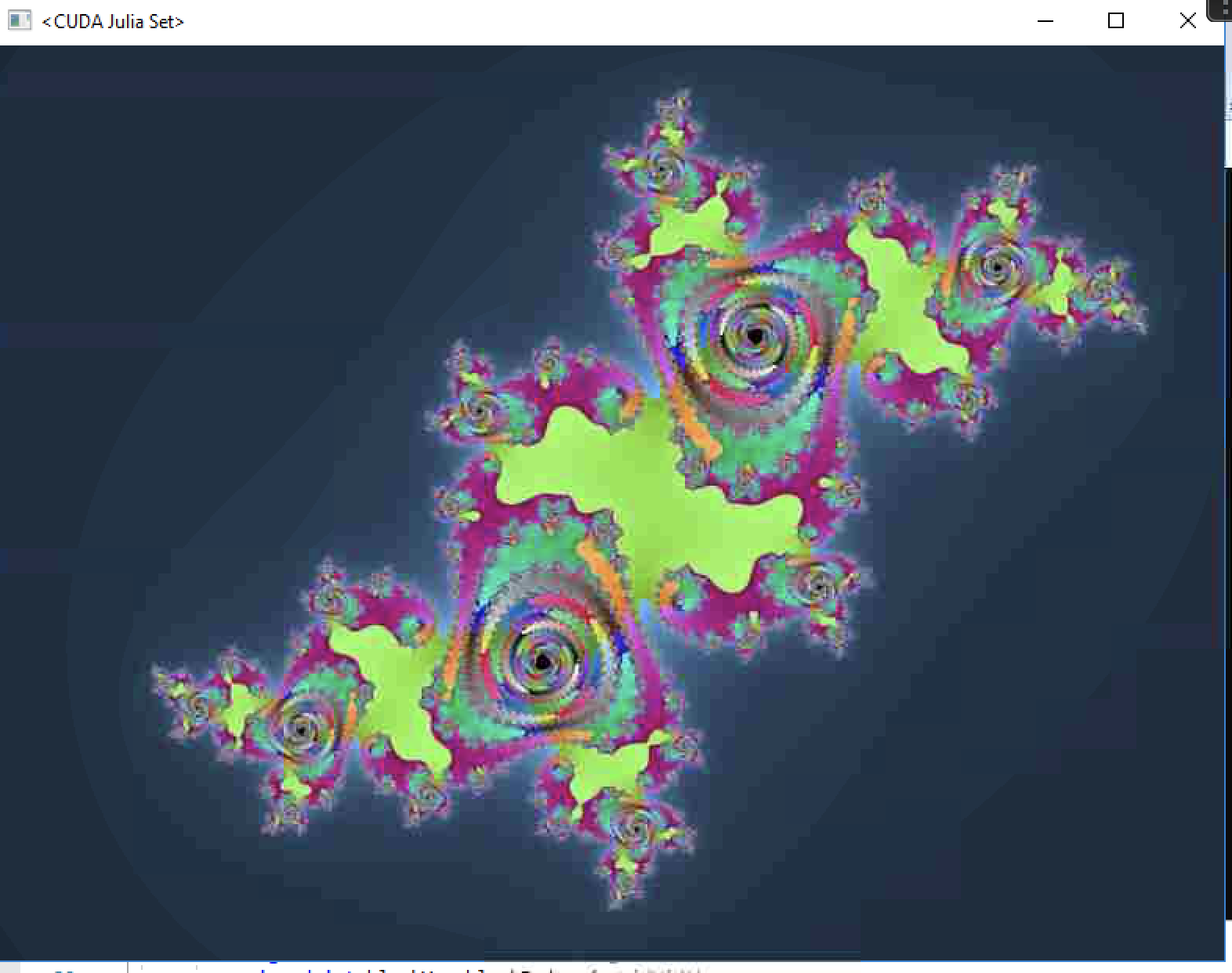
Imaging examples
The image denoising project in the “3_Imaging” folder is a good example that highlights the GPU roots, using the parallel capability to handle images.
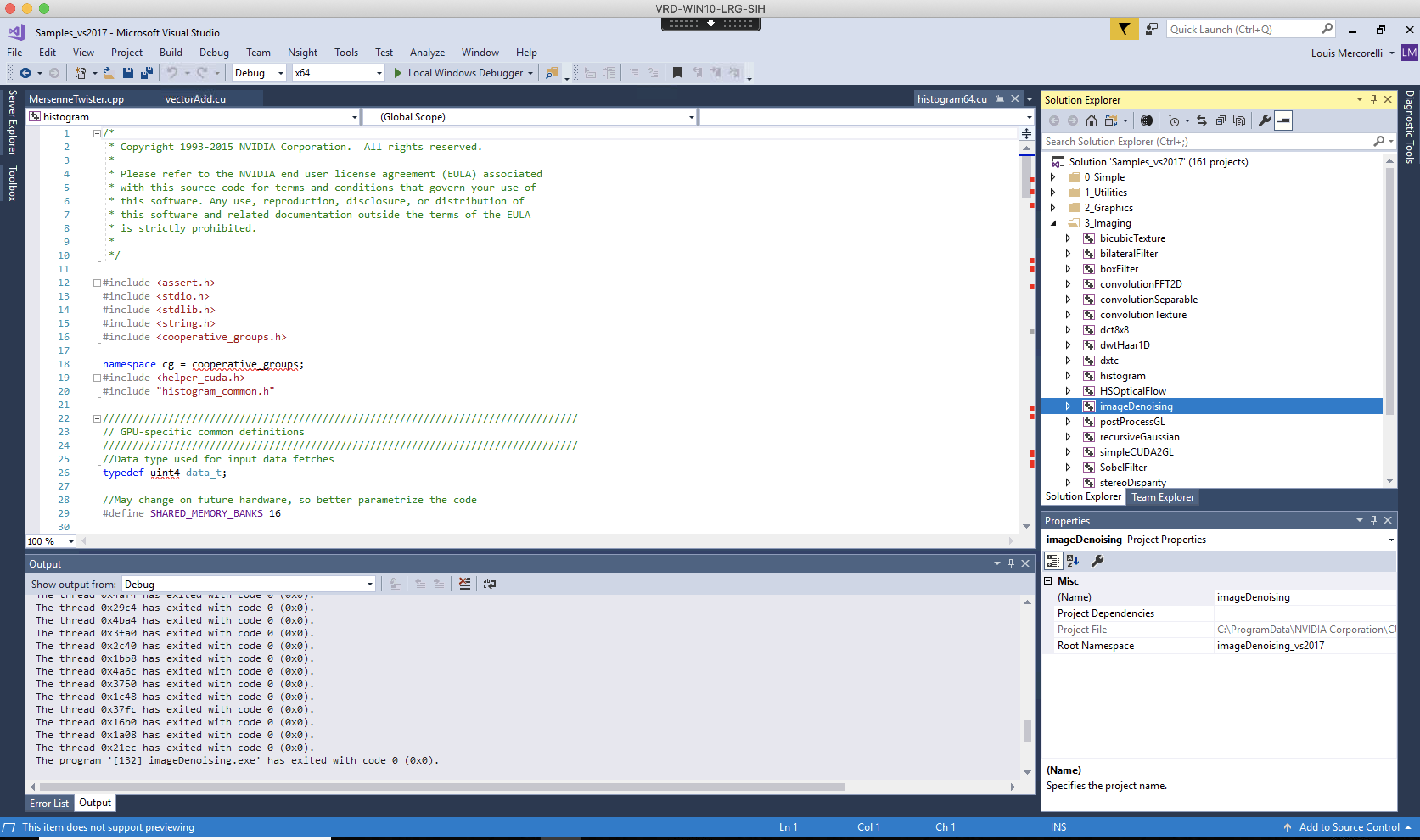
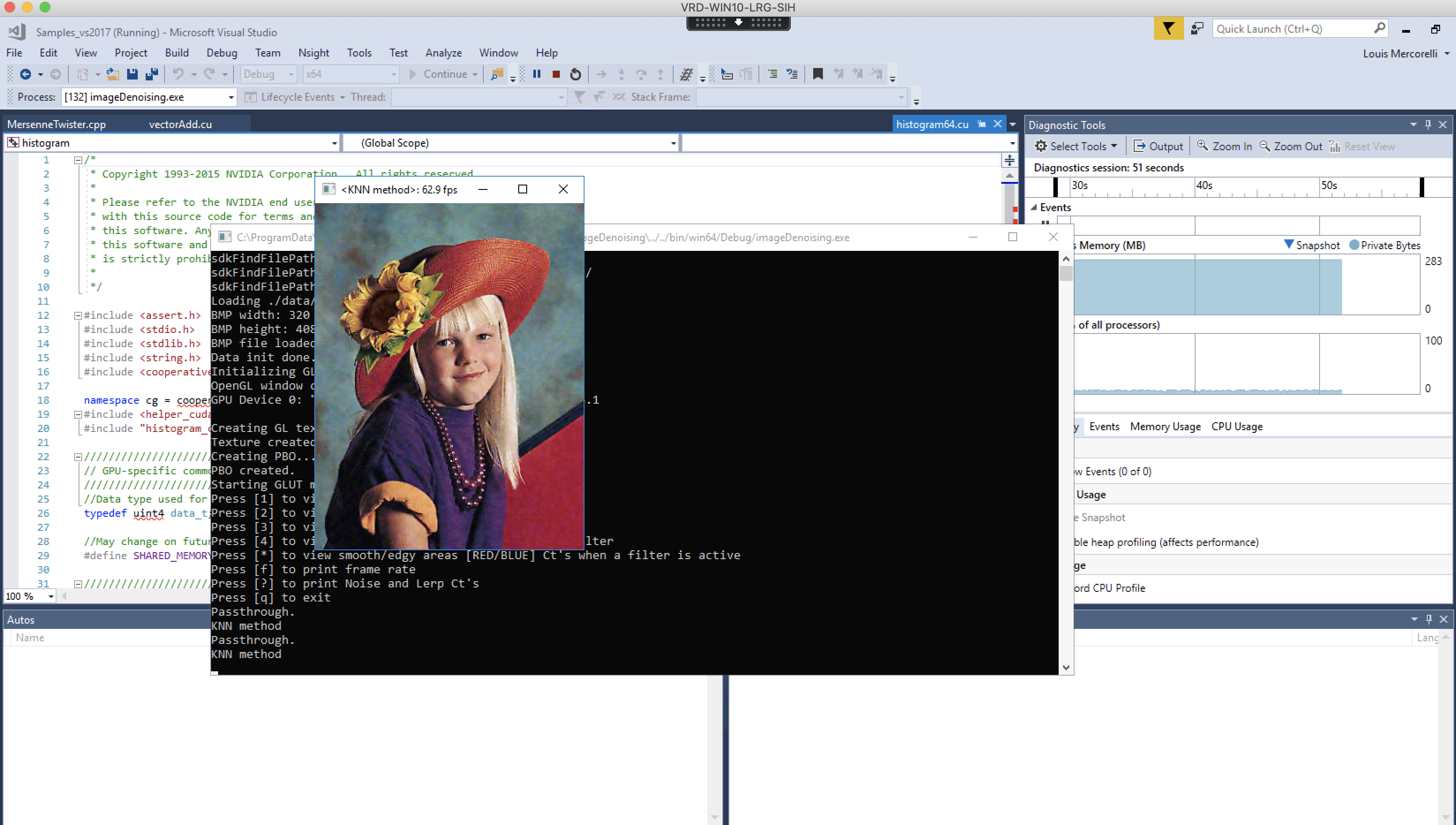
Simulation examples
The smoke particles simulation in the “5_simulations” folder is a nice example of the strength of a GPU with 3-D simulations. Notice the shadows, blurring and particle dispersion all handled via calculations done by the GPU in parallel. A still image can be seen below but running the module is obviously more impressive.
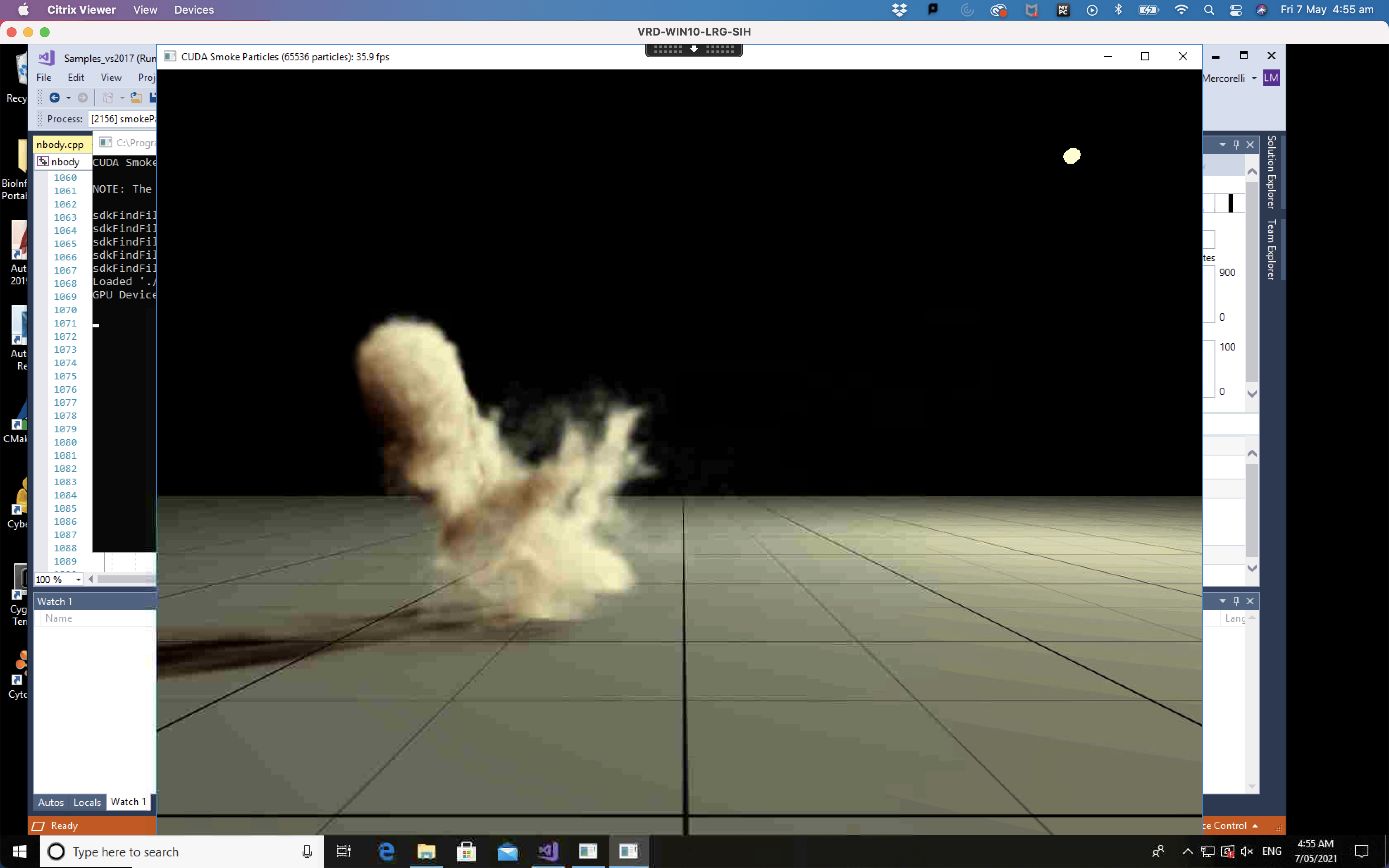
Other examples
There are over 150 examples in the CUDA sample directory. Finding one that perfectly matches your needs is impossible but it isn’t difficult to find something close for a great starting point.
We recommend trying out a few on your own. Running them is fun but making small changes to them is the best way to really understand CUDA and GPUs. Good luck and HAPPY PARALLEL programming…
… WAIT before you finish up don’t forget the last section, how to CORRECTLY logoff of an Argus Win10 machine.
Key Points
There are a lot of CUDA examples but sometimes your GPU will not support all the functionality
Not all GPUs are compliant with all software